MIT 18.S191 | Spring 2021 | Notes
Contents
Note on Course: Introduction to Computational Thinking
Computational science can be summed up by a simplified workflow:
data
==>input
==>process
==>model
==>visualize
==>output
Topics Include:
- Image Analysis
- Particle Dynamics and Ray Tracing
- Epidemic Propagation
- Climate Modeling
Module 1: Images
1. Install Julia and Pluto
- Step 1: Download from https://julialang.org/downloads/
- Step 2: Open Julia and Install Pluto
- To install Pluto, we want to run a package manager command. To switch from Julia mode to Pkg mode, type
]
(closing square bracket)
- To install Pluto, we want to run a package manager command. To switch from Julia mode to Pkg mode, type
- Step 3:
import Pluto
- Step 4:
Pluto.run()
|
|
2. Images as Data and Arrays
2.1. Pixel
If we open an image on our computer or the web and zoom in enough, we will see that it consists of many tiny squares, or pixels (“picture elements”).
- Each pixel is a block of one single colour
black = RGB(0, 0, 0)
red = RGB(1, 0, 0)
green = RGB(0, 1, 0)
blue = RGB(0, 0, 1)
- and the pixels are arranged in a two-dimensional square grid.
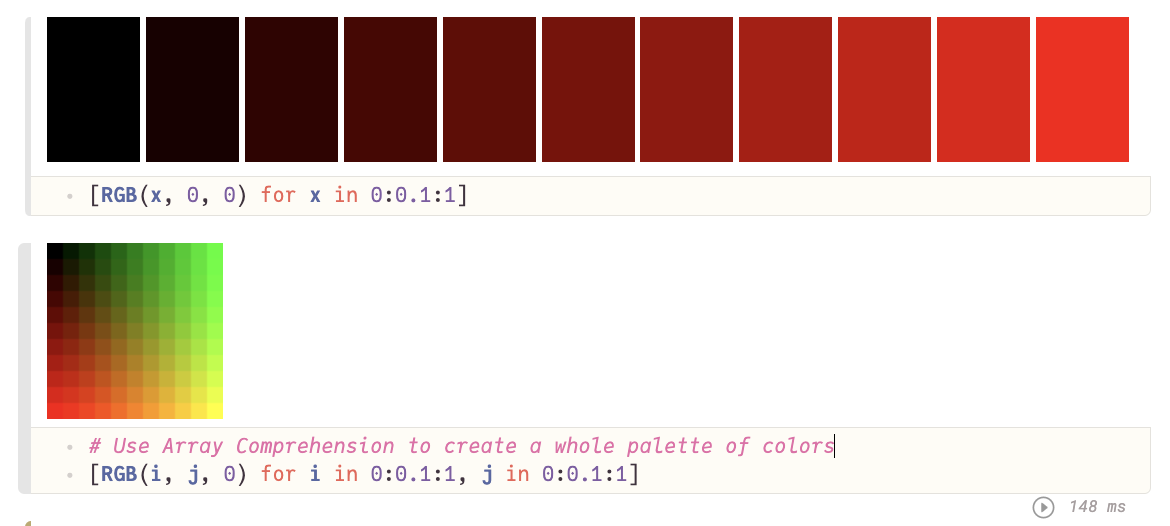
Note that an image is already an approximation of the real world
- it is a two-dimensional, discrete representation of a 3D reality.
Interactivity using Sliders
The Slider
type is defined in the PlutoUI.jl
package.
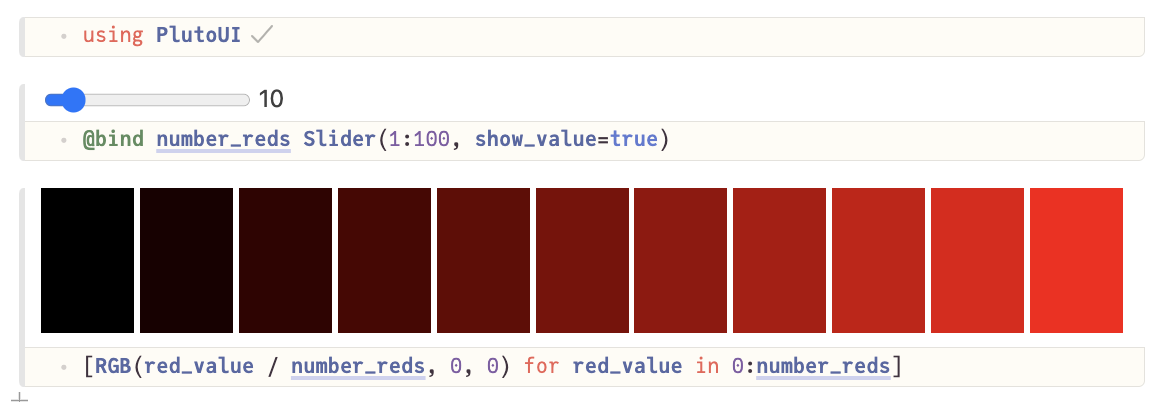
2.2. Download and Load 2D Images
|
|
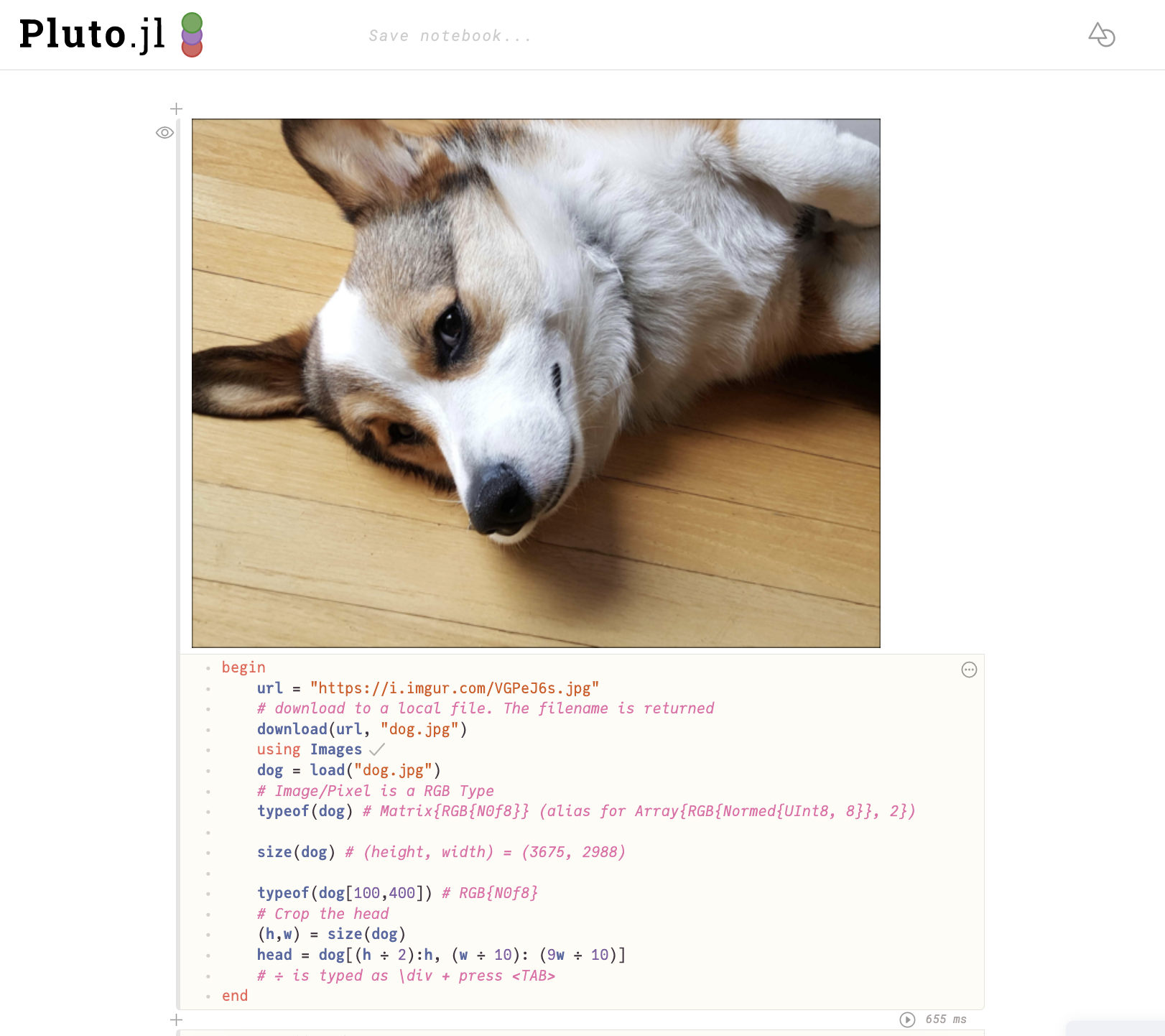
2.3. Images Flip and Concatentaion as Matrix
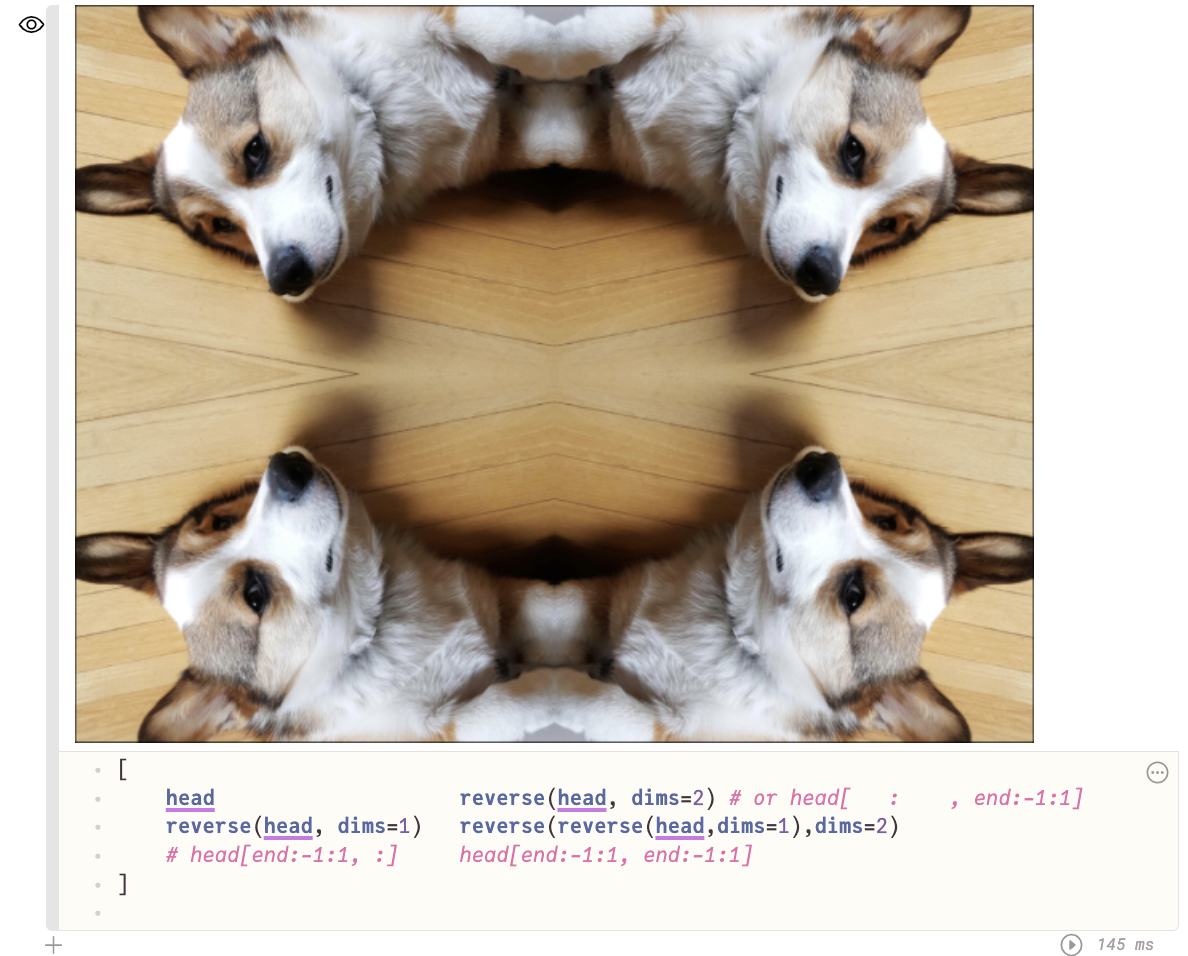
2.4. Locate and Modify Pixels
|
|
- This creates a new variable called
number_reds
, whose value is the value shown by the slider. When we move the slider, the value of the variable gets updated. Pluto is a reactive notebook, other cells which use the value of this variable will automatically be updated too!